With the
improvement in technology, the trends of programming languages have shifted
towards the Object Oriented Programming(OOP). Here I tried to explain classes
and Objects in java.
Object oriented programming
means any kind of programming language which uses some object oriented
concepts. In OOP, programmer not only define the data types of data structure
but also the functions to be performed. In this way, data structure becomes an
object which includes data and function. Examples of Object oriented
languages are C++, Java etc.
Main concepts of OOP
Some of the main concepts of Object Oriented
Programming are:
1.
Classes
2.
Data Abstraction
3.
Information Hiding
4.
Inheritance
5.
Polymorphism
6.
Encapsulation
Java classes
I will explain the concept
of classes in java here. A class is a blueprint or prototype from which objects
are created. This section defines a class that models the state and behavior of
a real-world object. It intentionally focuses on the basics, showing how even a
simple class can cleanly model state and behavior. A class contain data members
and functions. A class defines all the common properties of object that belong
to it.
Example of class in
java
For example you want to write a class of car
in java. As I stated earlier class is simply a prototype of real world object.
So first think about the car for which you have to write a class.
A car has some properties, like car is of red color,
car has some price and other in the same way. These properties are data members
for which you have to write functions or operations or some action of real
world. Like if you want to know car color, you have to write a function which
will return color of the cars. That's it. Here a sample of class is given
below:
public class car{
String color;
double price;
String model;
String getCarColor(){
}
double getCarPrice(){
}
}
Defining a class
Variable types in a class
A class can contain any of the following variable types.
- Local variables: Variables defined inside methods, constructors or blocks are called local variables. The variable will be declared and initialized within the method and the variable will be destroyed when the method has completed.
- Instance variables: Instance variables are variables within a class but outside any method. These variables are initialized when the class is instantiated. Instance variables can be accessed from inside any method, constructor or blocks of that particular class.
- Class variables: Class variables are variables declared with in a class, outside any method, with the static keyword.
A class can have any number of methods to access the value of various kinds of methods. In the above example, barking(), hungry() and sleeping() are methods.
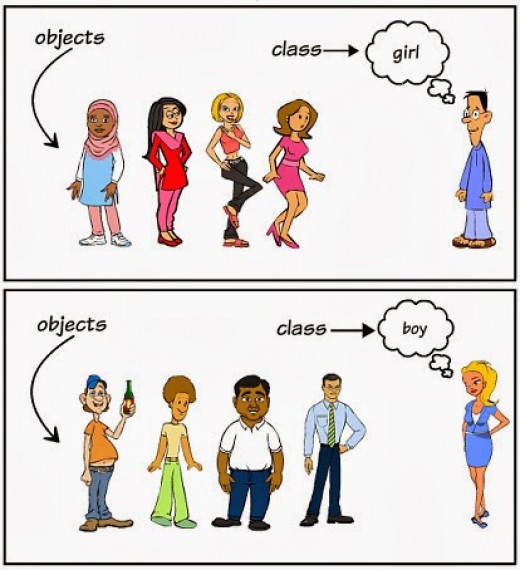
No comments:
Post a Comment